Lightning Web Components (LWC) is a powerful tool for building web applications in the Salesforce ecosystem. With LWC, developers can create reusable and modular components that can be easily combined to create complex apps. However, to achieve this level of complexity, effective communication between LWC components is essential. Without it, components cannot pass data between each other or respond to user events. This article will explore the different ways to achieve communication between LWC components, providing you with the knowledge and skills to create efficient and scalable solutions.
Types of Communication between LWC Components:
In LWC, there are three main types of communication patterns: parent to child, child to parent, and sibling to sibling. Each pattern serves a unique purpose and requires a different approach to implement effectively. In this section, we will explore each type of communication pattern and provide you with the knowledge you need to enable effective communication between your LWC components.
Parent to child communication in LWC
Parent to child communication is a communication pattern in LWC where a parent component passes data or triggers an action in one or more child components. This communication pattern is essential in LWC since it allows developers to create reusable components that can be easily combined to create complex applications.
For example, a parent component might pass data to a child component such as a list of records, which the child component then displays in a table. Alternatively, the parent component might trigger an action in a child component when a user interacts with a button or other UI element. By enabling parent to child communication, you can create highly interactive and dynamic applications that respond to user input in real-time.
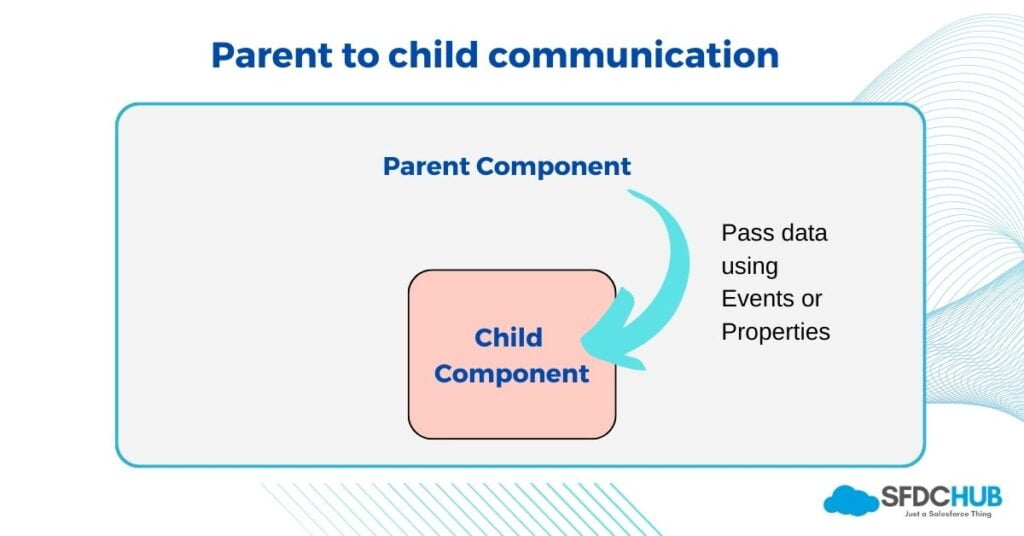
There are several ways to achieve parent to child communication. Each method has its strengths and weaknesses, and choosing the right approach depends on the specific requirements of your application. In this section, we will discuss the different ways to achieve parent to child communication in LWC, including using component properties, and events. We will explore the pros and cons of each method and provide you with the knowledge you need to choose the right approach for your application.
Parent to child communication using Properties
One way to achieve parent to child communication in LWC is by passing data through component properties. This method involves defining a property on the child component and then setting its value from the parent component. The child component can then access the value of the property and use it to display data or trigger an action. Here’s an example:
Parent Component HTML:
<template>
<c-child-component message={message}></c-child-component>
</template>
Parent Component JS:
import { LightningElement } from 'lwc';
export default class ParentComponent extends LightningElement {
message = 'Hello World!';
}
Child Component JS:
import { LightningElement, api } from 'lwc';
export default class ChildComponent extends LightningElement {
@api message;
connectedCallback() {
console.log(this.message); // Outputs "Hello World!" to the console
}
}
In this example, the parent component sets the value of the message
property on the child component by passing it as an attribute. The child component then accesses the value of the property using the @api
decorator and logs it to the console. This is a simple and effective way to pass data from a parent component to a child component in LWC.
Parent to child communication using events
Another way to achieve parent to child communication in LWC is by using events. This method involves defining a custom event on the child component and then dispatching it from the parent component. The child component can then handle the event and use the data passed in the event payload to update its state or trigger an action. Here’s an example:
Parent Component HTML:
<template>
<c-child-component onselected={handleSelected}></c-child-component>
</template>
Parent Component JS:
import { LightningElement } from 'lwc';
export default class ParentComponent extends LightningElement {
handleSelected(event) {
const recordId = event.detail.recordId;
console.log('Selected Record Id:', recordId);
}
}
Child Component JS:
import { LightningElement } from 'lwc';
export default class ChildComponent extends LightningElement {
handleSelect(event) {
const recordId = '001XXXXXXXXXXXXXXX'; // Placeholder for selected record Id
const selectedEvent = new CustomEvent('selected', { detail: { recordId }});
this.dispatchEvent(selectedEvent);
}
}
In this example, the child component defines a custom event called ‘selected’, which is dispatched from the child component when a record is selected. The parent component handles the event by defining a method called handleSelected
, which logs the record ID to the console.
Child to Parent communication in LWC
Child to parent communication in LWC refers to the process of passing data or events from a child component to a parent component in the component hierarchy. This allows child components to notify their parent components of important events, such as when a user interacts with the child component, or when the child component’s state changes.
By enabling child components to communicate with their parent components, developers can create more dynamic and interactive applications that respond to user actions in real-time. There are several ways to achieve child to parent communication in LWC, including using events, invoking parent component methods, and passing data using properties.
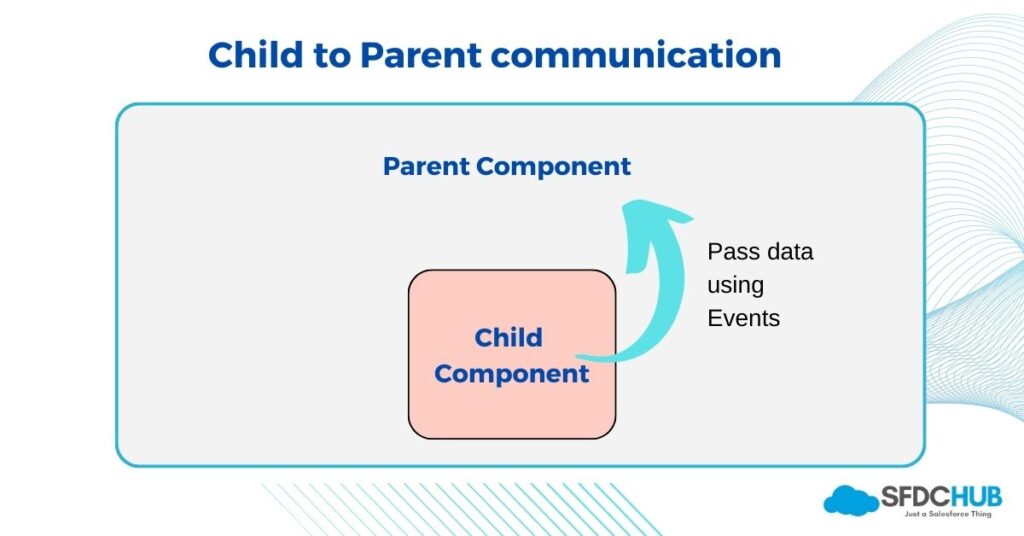
In LWC, there are several ways to achieve child to parent communication. The most common method is to use events, which allow child components to dispatch custom events that parent components can listen for and respond to. Events can be dispatched by child components using the CustomEvent
constructor, and can include data that is passed from the child to the parent.
Additionally, child components can pass data to parent components using properties, which can be defined on the child component and accessed by the parent component. By understanding these different approaches to child to parent communication in LWC, developers can choose the method that best suits their application’s requirements and design effective component hierarchies that facilitate communication between components.
Child to Parent communication using events
Child to parent communication using events is another common method in LWC. This method involves the child component dispatching a custom event that the parent component listens for and responds to. Here’s an example:
Child Component HTML:
<template>
<lightning-button label="Send Message" onclick={handleButtonClick}></lightning-button>
</template>
Child Component JS:
import { LightningElement } from 'lwc';
export default class ChildComponent extends LightningElement {
handleButtonClick() {
const messageEvent = new CustomEvent('message', {
detail: { text: 'Hello from child component' }
});
this.dispatchEvent(messageEvent); // Dispatches the custom event
}
}
Parent Component HTML:
<template>
<c-child-component onmessage={handleMessage}></c-child-component>
</template>
Parent Component JS:
import { LightningElement } from 'lwc';
export default class ParentComponent extends LightningElement {
handleMessage(event) {
console.log('Message Received:', event.detail.text); // Logs the message to the console
}
}
In this example, the child component dispatches a custom event called “message” when the button is clicked. The parent component listens for this event using the onmessage
attribute and handles it by logging the message to the console. This method of child to parent communication using events is particularly useful for enabling child components to notify their parent components of important events, such as when a user interacts with the child component or when the child component’s state changes. It can be used to build more dynamic and interactive applications in LWC.
Sibling to sibling communication in LWC
In addition to communication between parents and children and between children and parents, Lightning Web Components also support communication between sibling components, which is known as sibling to sibling communication. Sibling to sibling communication is used when two or more components on the same level of the component hierarchy need to communicate with each other. In this section, we will discuss how to achieve sibling to sibling communication in LWC and explore some of the different methods available.
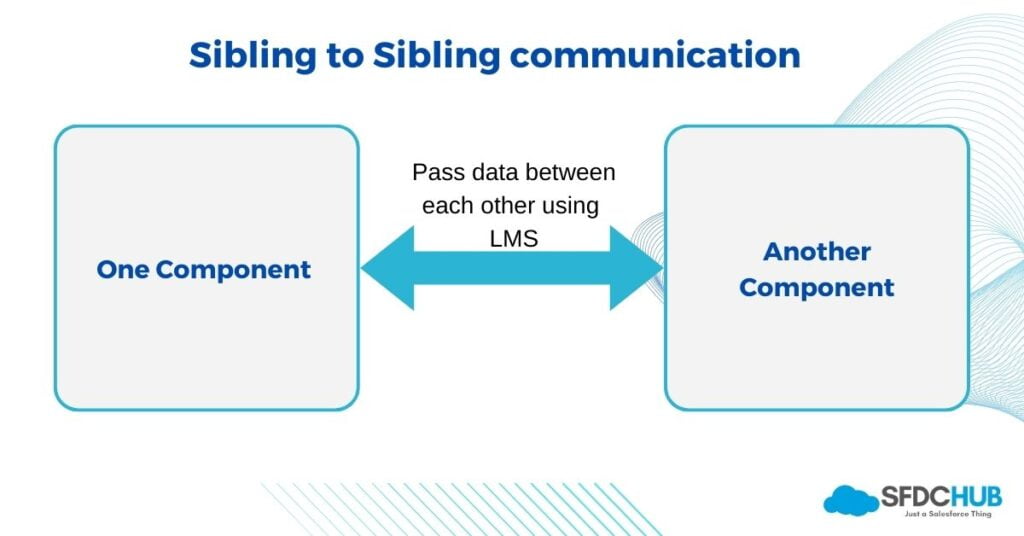
There are several ways to achieve sibling to sibling communication in Lightning Web Components. One method is to use a shared service, where each sibling component accesses a common service to share data or trigger actions. Another method is to use a higher-level component as a mediator or hub to relay messages or events between sibling components.
Additionally, sibling components can also communicate directly with each other using the Lightning Message Service or by using custom events. Each method has its own advantages and trade-offs, and the best approach will depend on the specific requirements of the use case. In the following sections, we will explore each of these methods in more detail and provide examples of how they can be used to achieve sibling to sibling communication in LWC.
Lightning Message Service in LWC
In LWC, the Lightning message service provides a powerful way for sibling components to communicate with each other. The message service is based on the publish-subscribe pattern, where components can publish messages and other components can subscribe to receive those messages.
To use the message service, you need to define a message channel that the publisher and subscriber components can use to communicate. The channel defines the type of message and any data that needs to be passed between components.
Here’s an example of how to use the Lightning message service for sibling to sibling communication:
1. Define a message channel in a separate module:
import { LightningElement } from 'lwc';
import { createMessageChannel } from 'lightning/messageService';
export default class MyMessageChannel extends LightningElement {
connectedCallback() {
// Define the message channel
this.messageChannel = createMessageChannel();
}
}
2. In the publishing component, import the message channel and publish the message:
import { LightningElement, wire } from 'lwc';
import MY_MESSAGE_CHANNEL from '@salesforce/messageChannel/MyMessageChannel__c';
import { publish, MessageContext } from 'lightning/messageService';
export default class PublisherComponent extends LightningElement {
@wire(MessageContext)
messageContext;
handleClick() {
// Publish the message with data
const message = {
value: 'Hello from publisher!'
};
publish(this.messageContext, MY_MESSAGE_CHANNEL, message);
}
}
3. In the subscribing component, import the message channel and subscribe to receive the message:
import { LightningElement, wire } from 'lwc';
import MY_MESSAGE_CHANNEL from '@salesforce/messageChannel/MyMessageChannel__c';
import { subscribe, MessageContext } from 'lightning/messageService';
export default class SubscriberComponent extends LightningElement {
@wire(MessageContext)
messageContext;
connectedCallback() {
// Subscribe to receive the message
this.subscription = subscribe(
this.messageContext,
MY_MESSAGE_CHANNEL,
(message) => {
// Handle the message
console.log('Received message:', message.value);
}
);
}
disconnectedCallback() {
// Unsubscribe when the component is removed from the DOM
unsubscribe(this.subscription);
}
}
In this example, the publishing component sends a message with the value ‘Hello from publisher!’ when the handleClick() method is called. The subscribing component receives the message and logs the value to the console.
The Lightning message service provides a flexible and scalable way for components to communicate with each other, making it a powerful tool for building complex applications with LWC.
Conclusion
In conclusion, communication between LWC components is essential to building powerful, interactive, and dynamic web applications. This article has discussed various ways to achieve communication between different types of components in LWC. From parent-child, child-parent to sibling-sibling communication, developers have a wide range of techniques to choose from depending on their requirements.
It is crucial to choose the right technique to ensure efficient and effective communication between components. By following the examples and guidelines provided in this article, developers can create well-structured and maintainable code that allows different components to communicate seamlessly, resulting in a more robust and scalable application.
References
Also Read:
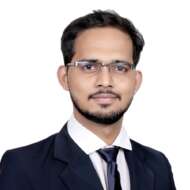
Abhishek
Mr. Abhishek, an experienced Senior Salesforce Developer with over 3.5+ years of development experience and 6x Salesforce Certified. His expertise is in Salesforce Development, including Lightning Web Components, Apex Programming, and Flow has led him to create his blog, SFDC Hub.