One of the key features of LWC is the ability to use decorators to extend the functionality of components. Decorators in Lightning Web Components are a way to add metadata and behavior to a component’s properties and methods, without modifying the underlying code. In this article, we’ll explore the different types of decorators in LWC. We’ll also see some common use cases for decorators and some limitations and restrictions. By the end of this article, we should have a solid understanding of how to use decorators to create powerful, flexible, and reusable components.
Types of Decorators in LWC
@api decorator
In LWC, an API decorator is used to expose a public property or method in a component, which can then be accessed and used by other components or code.
Let’s take a look at an example of how to use the @api decorator in Lightning Web Components:
import { LightningElement, api } from 'lwc';
export default class ExampleComponent extends LightningElement {
@api greeting = 'Hello';
handleClick() {
console.log('Button clicked!');
}
}
In this example, we have a component named “ExampleComponent” which has a public property with the name “greeting”. The @api decorator is used to make this property available for use by other components.
Other components can then access the “greeting” property like this:
<template>
<c-example-component greeting="Hi there!"></c-example-component>
</template>
In this example, we are setting the “greeting” property to “Hi there!” when we use the ExampleComponent in our template.
Additionally, we can also use the @api decorator on methods to make them public and callable from other components. Let’s take a look at an example:
import { LightningElement, api } from 'lwc';
export default class ExampleComponent extends LightningElement {
@api
handleClick() {
console.log('Button clicked!');
}
}
In this example, we have a public method called “handleClick” that can be called from other components. Other components can call this method like this:
<template>
<c-example-component onbuttonclick={handleButtonClick}></c-example-component>
</template>
In this example, we are setting the “onbuttonclick” attribute to a method called “handleButtonClick” defined in the parent component. This will allow the parent component to handle the button click event using the child component’s public “handleClick” method.
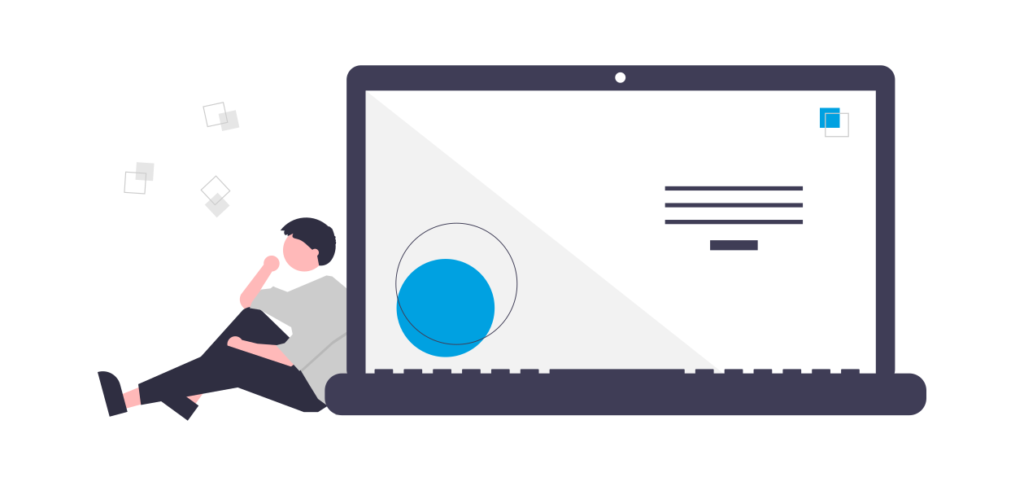
@track decorator
In LWC, the @track decorator is used to track changes to a property or object and update the component’s UI accordingly.
Let’s see an example of how to use the @track decorator in Lightning Web Components:
import { LightningElement, track } from 'lwc';
export default class ExampleComponent extends LightningElement {
@track greeting = 'Hello';
@track person = { name: 'John', age: 30 };
handleClick() {
this.greeting = 'Hi';
this.person.age = 31;
}
}
In this example, we have a component “ExampleComponent” which has two tracked properties: “greeting” and “person”.
Whenever the value of a tracked property changes, the component’s UI will automatically update to reflect the new value. In the example above, the UI would update whenever the “greeting” property is changed or when any property of the “person” object is changed.
We can also use the @track decorator on variables to make them reactive, like so:
import { LightningElement, track } from 'lwc';
export default class ExampleComponent extends LightningElement {
@track count = 0;
handleIncrement() {
this.count++;
}
handleDecrement() {
this.count--;
}
}
In this example, we have a “count” variable that is being tracked using the @track decorator. Whenever the “count” variable is incremented or decremented in the methods, the UI will automatically update to show the new value.
Note that the @track decorator is not required for primitive data types like strings or numbers, but is required for objects and arrays to track changes to their properties or elements.
The @track decorator was used to marking a property as reactive, which means that changes to the property will trigger a re-render of the component. But it was changed after Sprint 20 release. Now, there is no need to use @track decorator.
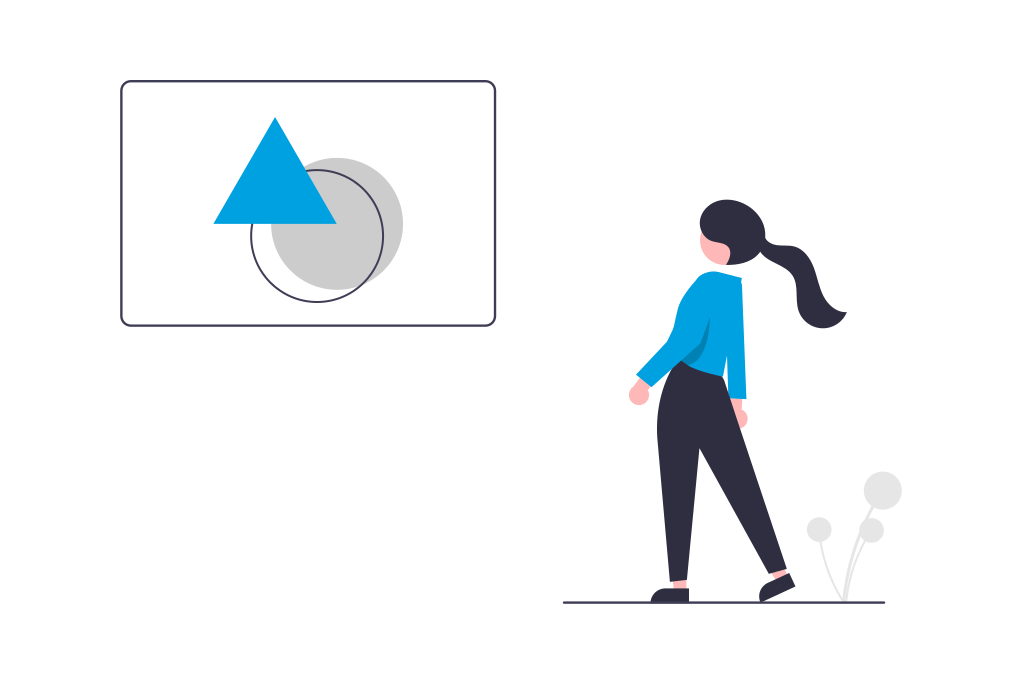
@wire decorator
The wire decorator is used to connect a component’s properties or methods to data from an Apex controller or other data source. Let’s see an example of how to use the wire decorator to fetch a list of accounts from Salesforce Org and display them in an LWC component:
import { LightningElement, wire } from 'lwc';
import getAccounts from '@salesforce/apex/AccountController.getAccounts';
export default class AccountList extends LightningElement {
accounts;
@wire(getAccounts)
wiredAccounts({ error, data }) {
if (data) {
this.accounts = data;
} else if (error) {
console.error(error);
}
}
}
In this example, we’re using the @wire
decorator to connect the getAccounts
method in our Apex controller to the wiredAccounts
method in our LWC component. The getAccounts
method returns a list of accounts, which we’re storing in the accounts
property of our component. If there’s an error when fetching the accounts, we log the error to the console.
When the component is first rendered, the wiredAccounts
method is called automatically and the getAccounts
method is invoked to fetch the data. Once the data is returned, it’s stored in the accounts
property and the component is re-rendered to display the updated data.
Using the wire decorator is a powerful way to fetch data from a server and display it in an LWC component, without the need for complex callbacks or custom event handlers. By using the wire decorator, we can easily connect our components to data sources and keep our code simple and concise.
Conclusion
In conclusion, decorators in LWC provide a powerful way to extend functionality. The “@api” decorator allows us to expose properties and methods of our component to be used in parent components. The “@track” decorator enables the reactive rendering of data by tracking changes to properties, while the “@wire” decorator allows us to retrieve data from Apex controllers and other sources in an efficient manner.
Overall, decorators are an important feature to understand and utilize when working with Lightning web components.
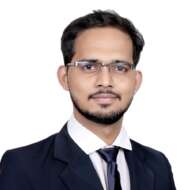
Abhishek
Mr. Abhishek, an experienced Senior Salesforce Developer with over 3.5+ years of development experience and 6x Salesforce Certified. His expertise is in Salesforce Development, including Lightning Web Components, Apex Programming, and Flow has led him to create his blog, SFDC Hub.