Lightning Web Components (LWC) is a modern web development framework that provides a range of features for building fast, efficient, and responsive web applications. Two important features of LWC are Wire Service and Imperative calls. Wire Service is a data binding feature that fetches data from various sources, such as Apex methods, Lightning Data Service, and custom adapters.
Imperative calls, on the other hand, provide a way to make direct calls to Apex methods from your LWC component. Understanding the difference between these two features is essential for building efficient and effective LWC components. In this article, we’ll dive into the concepts of Wire Service and Imperative calls, explore their differences and use cases, and provide practical examples to help you get started with these powerful features.
Introduction to Wire Services in LWC
Wire Services are an essential part of Lightning Web Components (LWC), providing a declarative way to fetch data and properties from Apex controllers, JavaScript controllers, and other data sources in your components. Wire Services are based on a reactive approach, where components are updated automatically as the data changes, making it easier to build dynamic and responsive user interfaces.
So why are Wire Services so important? For starters, they provide a more efficient way to fetch data and properties, reducing the amount of boilerplate code you need to write. By using Wire Services, you can fetch data and properties with just a few lines of code, making your components more readable and easier to maintain. Additionally, Wire Services help to improve the performance of your components by automatically managing the caching of data and only fetching what is necessary.
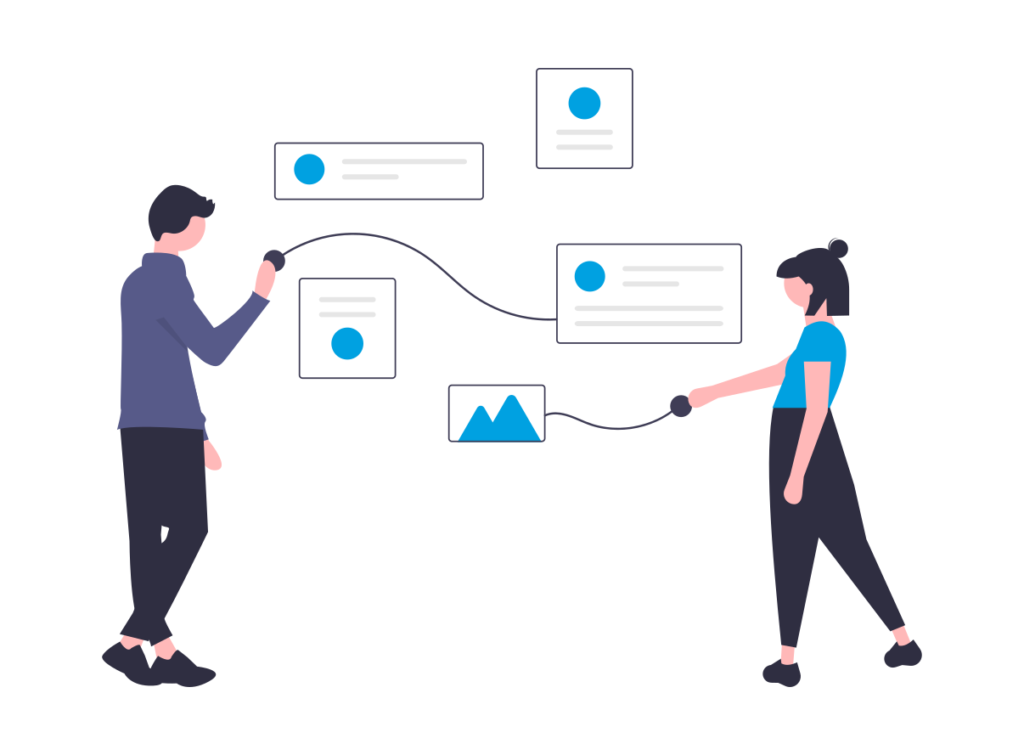
Wire Services are a key feature of LWC that differentiates it from other web frameworks. They make it easier to develop complex applications with reusable components and enable developers to focus on building great user experiences instead of worrying about data fetching and caching.
In this post, we’ll dive into how to create and use Wire Services in LWC, including best practices and advanced topics. By the end, you’ll have a deep understanding of how Wire Services work and how to use them to build better LWC components.
How can you create Wire Services in LWC?
To create a Wire Service in LWC, you’ll need to define a JavaScript function that fetches data or properties from a data source. This function should use the @wire
decorator to indicate that it’s a Wire Service, and specify the properties that it needs to fetch using the wire
adapter.
The syntax of a Wire Service function looks like this:
import { LightningElement, wire } from 'lwc';
import getContacts from '@salesforce/apex/ContactController.getContacts';
export default class ContactList extends LightningElement {
@wire(getContacts) contacts;
}
In this example, we’re using the @wire
decorator to create a Wire Service that fetches contacts from an Apex controller method called getContacts
. The contacts
property is then populated with the data returned by the method, which can be used in the component’s template.
Points to Note in Wire Services
- Wire Services should always be used for asynchronous data retrieval. Synchronous calls can result in poor performance and even block the UI.
- Use the
@wire
decorator to bind a function or property to a data source. This allows LWC to manage the data and update the UI automatically. - When using Function Wire Services, use the
@wire
decorator to bind a JavaScript function to a property in your component. The function should call an Apex method and return the data. - When using Property Wire Services, use the
@wire
decorator to bind a property to a data source, such as a Lightning Data Service or Apex method. The property should reflect the current state of the data. - Use dynamic binding to bind parameters to Function Wire Services. This allows the function to be called whenever the parameter values change.
- Use error handling to handle errors that may occur when using Wire Services gracefully. LWC provides a way to catch and handle errors using the
error
property on the result object. - Avoid calling multiple Wire Services that fetch data from the same source. This can result in redundant data retrieval and poor performance.
- Always check the data and errors returned from Wire Services before using them in your component. This helps prevent errors and undefined behavior.
- When working with data lists, use the
Array.isArray()
method to check if the data is an array before accessing it. This helps prevent errors when the data is not in the expected format.
What are Imperative calls in LWC?
Imperative calls in LWC are used to make an asynchronous request to a server using JavaScript code. Unlike Wire Services, imperative calls do not use the @wire
decorator to automatically manage data and update the UI.
To make an imperative call, you can use the import
statement to import the required Apex method, then call the method using JavaScript code. Here’s an example:
import { LightningElement, track } from 'lwc';
import getAccountList from '@salesforce/apex/AccountController.getAccountList';
export default class AccountList extends LightningElement {
@track accounts;
connectedCallback() {
this.loadAccounts();
}
loadAccounts() {
getAccountList()
.then(result => {
this.accounts = result;
})
.catch(error => {
// Handle error
});
}
}
In this example, the getAccountList()
method is imported using the @salesforce/apex
syntax, which allows LWC to access the Apex method from the server. The loadAccounts()
method is then called when the component is connected to the DOM, which uses the getAccountList()
method to asynchronously retrieve a list of accounts from the server.
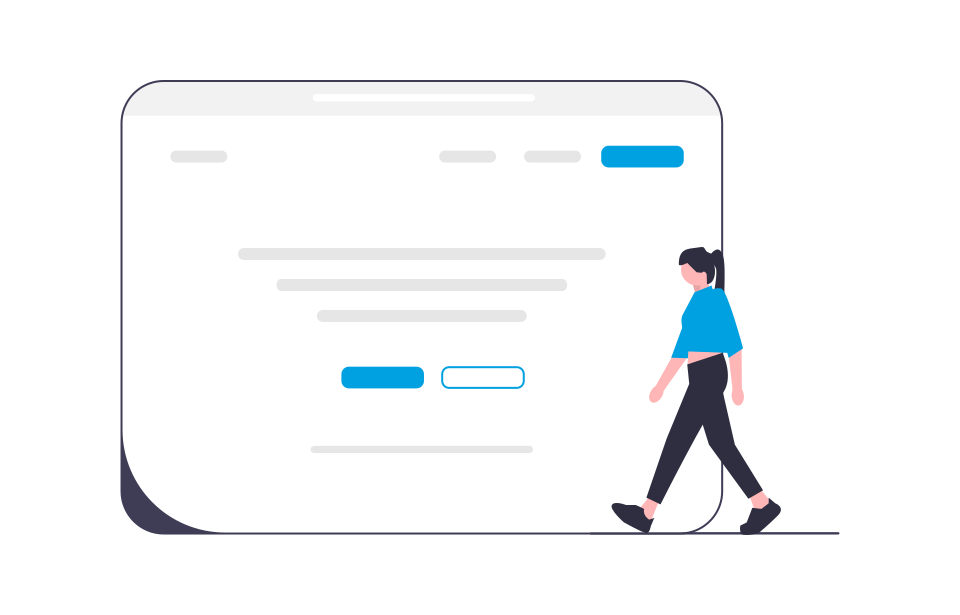
Once the data is retrieved, the then()
method is called with the result, which updates the accounts
property in the component using the @track
decorator. If an error occurs, the catch()
method is called, which allows you to handle the error and provide a user-friendly message.
Overall, Imperative calls are useful when you need more control over the data retrieval process or when you need to call an Apex method that takes input parameters. However, they require more code and do not offer the automatic data management and UI updates provided by Wire Services.
Points to Note in Imperative Calls
- Use Imperative calls when you need more control over the data retrieval process, such as when you need to pass input parameters to an Apex method.
- Unlike Wire Services, Imperative calls do not use the
@wire
decorator to automatically manage data and update the UI. You need to manually handle the data and UI updates in your JavaScript code. - Use the
import
statement to import the required Apex method, then call the method using JavaScript code. - Use Promises to handle asynchronous responses from the server. You can use the
then()
method to handle a successful response and thecatch()
method to handle errors. - Use the
@track
decorator to track changes to properties that are updated asynchronously. This allows LWC to re-render the UI when the data changes. - Use error handling to gracefully handle errors that may occur when using Imperative calls. You can use the
catch()
method to catch and handle errors. - Avoid calling multiple Imperative calls that fetch data from the same source. This can result in redundant data retrieval and poor performance.
- Always check the data and errors returned from Imperative calls before using them in your component. This helps prevent errors and undefined behavior.
- When working with data lists, use the
Array.isArray()
method to check if the data is an array before accessing it. This helps prevent errors when the data is not in the expected format.
Conclusion
In conclusion, both Wire Services and Imperative calls are powerful features of Lightning Web Components that allow you to retrieve data from a server and update the UI. Wire Services are ideal for simple data retrieval scenarios where automatic data management and UI updates are desired, while Imperative calls are useful when more control over the data retrieval process is required, or when input parameters need to be passed to the server.
When using Wire Services, it is important to understand the data binding and caching mechanisms that are used to optimize performance. You should also follow best practices such as using the appropriate data types, avoiding unnecessary data retrieval, and handling errors gracefully.
Similarly, when using Imperative calls, it is important to use Promises and error handling to manage asynchronous responses from the server and to avoid redundant data retrieval. Following these best practices will help ensure that your LWC components are efficient, maintainable, and provide a great user experience.
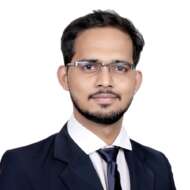
Abhishek
Mr. Abhishek, an experienced Senior Salesforce Developer with over 3.5+ years of development experience and 6x Salesforce Certified. His expertise is in Salesforce Development, including Lightning Web Components, Apex Programming, and Flow has led him to create his blog, SFDC Hub.